Operating Systems (CS111@UCSC) Projects Documentation
Shell
|---------- Source code here
The primary goal of this project was to become familiar with the system call interface and processes. A secondary goal was to use some of the programming tools provided in FreeBSD. A simple FreeBSD shell program was implemented that conveniently allows one to run other programs.
-
The internal shell command exit
Details: The exit command terminates the shell
Concepts: shell commands, exiting the shell
Library calls: exit() - A command with no arguments.
Example: ls
Details: This runs the command, which can be any executable in your default search path (the one that execvp() searches). Shell blocks until the command completes and, if the return code is abnormal (non-zero), a message is printed out.
Concepts: Forking a child process, waiting for it to complete, synchronous execution.
System calls: fork(), execvp(), wait() - A command with one or more arguments.
Example: ls -l foo
Details: Argument 0 is the name of the command; other arguments follow in sequence. A special character ends the command, which means that I/O redirection comes after all the arguments to a command.
Concepts: Command-line parameters
System calls: fork(), execvp(), wait() - A command, with or without arguments whose input is redirected from a file.
Example: sort -nr < scores
Details: This takes the named file as input to the command.
Concepts: Input redirection, file operations.
System calls: open(), close(), dup() - A command, with or without arguments, whose output is redirected to a file.
Example: ls -l > file
ls -l >> file
Details: This takes the output of the command and appends it to the named file. If the > form is used, the command fails if the file already exists. If the >> form is used, the command output is appended to the end of the output file, creating it if necessary. If the output file doesn't already exist, > and >> do the same thing.
Concepts: File operations, output redirection.
System calls: open(), close(), dup() - A command, with or without arguments, whose output is piped to the input of another command.
Example: ls -l | less
Details: This takes the output of the first command and makes it the input to the second command.
Concepts: Pipes, synchronous operation
System calls: pipe(), close(), dup() - Redirection of standard error.
Example: ls -l >& foo
ls -l >>& foo
ls -l |& wc
Details: If any output redirection operator includes an & character at the end, standard error (file descriptor 2) is redirected in addition to standard output, and both go to the same file / pipe.
Concepts: File operations, pipes, output redirection - System calls: pipe(), open(), close(), dup()
Two or more command sequences, possibly involving I/O redirection, separated by a semicolon.
Example: cd /home/manmeet ; sort -nr < names ; ls -l > foo
Details: The shell runs the first command, and waits for it to finish. When it finishes, the next command is run, regardless of any error encountered by the previous sequence. I/O redirections apply only to their specific command, not to all previous commands (or future commands).
Concepts: Multiple commands on a single line.
System calls: wait() - The internal shell command cd
Example: cd /usr
cd
Details: This shell command sets the working directory to the directory provided in the command. If no directory is provided, the working directory is set to whatever the working directory was when the shell was started
Concepts: Working directory
System / library calls: chdir(), getcwd()
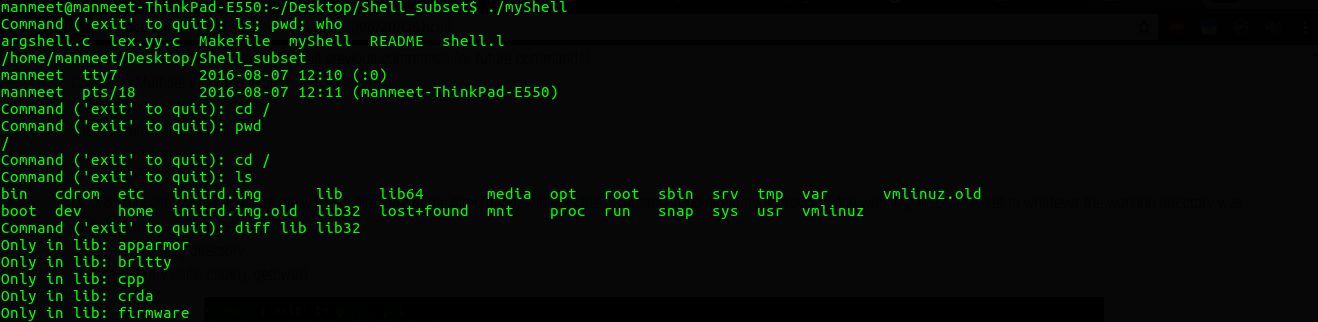
- - - - - - - - - - - - - - - - -
FAT File System
This was a file system to be accessed via FUSE. The entire disk is contained in a file in the underlying file system, and all of the operations written as routes to be called by the FUSE library.
Disk Structure
The disk is divided into 1KB blocks, each of which is read or written in its entirety. YThis is done using lseek() to seek to the appropriate offset (1024 * block_number), followed by a read() or write() of 1024 bytes. The blocks are laid out as follows:

The file allocation table (FAT) is an array of (signed) 4-byte block numbers. A 0 in the FAT means that a block is free, and a -2 means that the block is the last one in the file. Otherwise, the entry in the file allocation is the block number of the next block in the file. As noted above, the first k+1 entries of the FAT are invalid, and are set to -1 so they're never allocated.
Directories
Directory entries in this file system are 64 bytes long, and contain the following information: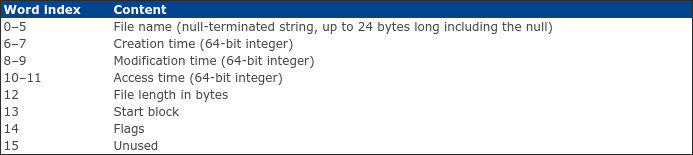